How To Use Web Components in React
Web Components enable us to develop entirely independently of frontend frameworks. Here is how to use them in React.
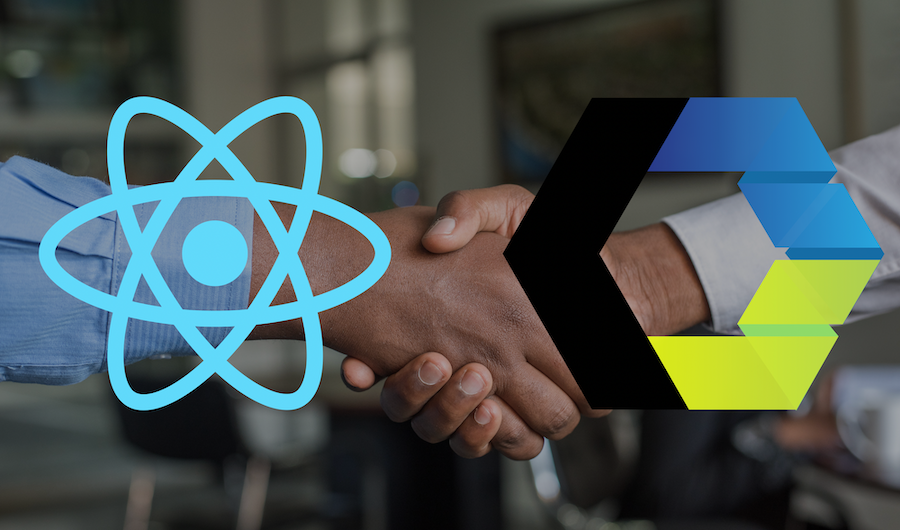
Many developers worry that Web Components might replace frontend frameworks and libraries.
In our eyes, that will not happen because they tackle different issues. The real key to a robust software architecture is combining them.
“Most people who use React don’t use Web Components, but you may want to […].” — https://reactjs.org
Before we dive into our example, let's look at the purpose of Web Components and React.
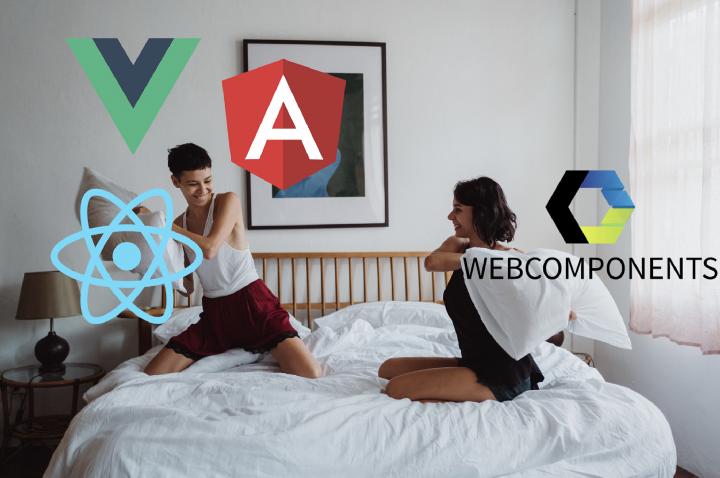
Purpose of Web Components
Web Components are reusable client-side components based on official web standards and supported by all major browsers. They are an excellent way of encapsulating functionality from the rest of our code. Not only that, but you can reuse them in every web application and web page.
Their purpose is to write strongly encapsulated custom elements to use everywhere. Web Components enable us to develop entirely independently of frontend frameworks.
The primary benefit of Web Components is that we can use them everywhere. With any framework, or even without a framework. — vuejs.org
It's really important to understand this point: Web Components are web standards that can be used anywhere and will always be relevant!
For example, when I started building my PDF & Web Highlighter Chrome Extension five years ago, I chose to create the core parts of my extension using Web Components.
That doesn't mean you should build everything with Web Components. It's completely fine to mix Web Components with React or any other frontend framework.
Over the years, I've switched out nearly every other technology—like moving from Vue 2 to Vue 3 and then from a client-side Vue app to Nuxt 3, among many other changes.
The one thing that has remained stable throughout it all is the Web Components, which have been with me since the start.
If you want to learn more about my tech stack, you can read more about it here:
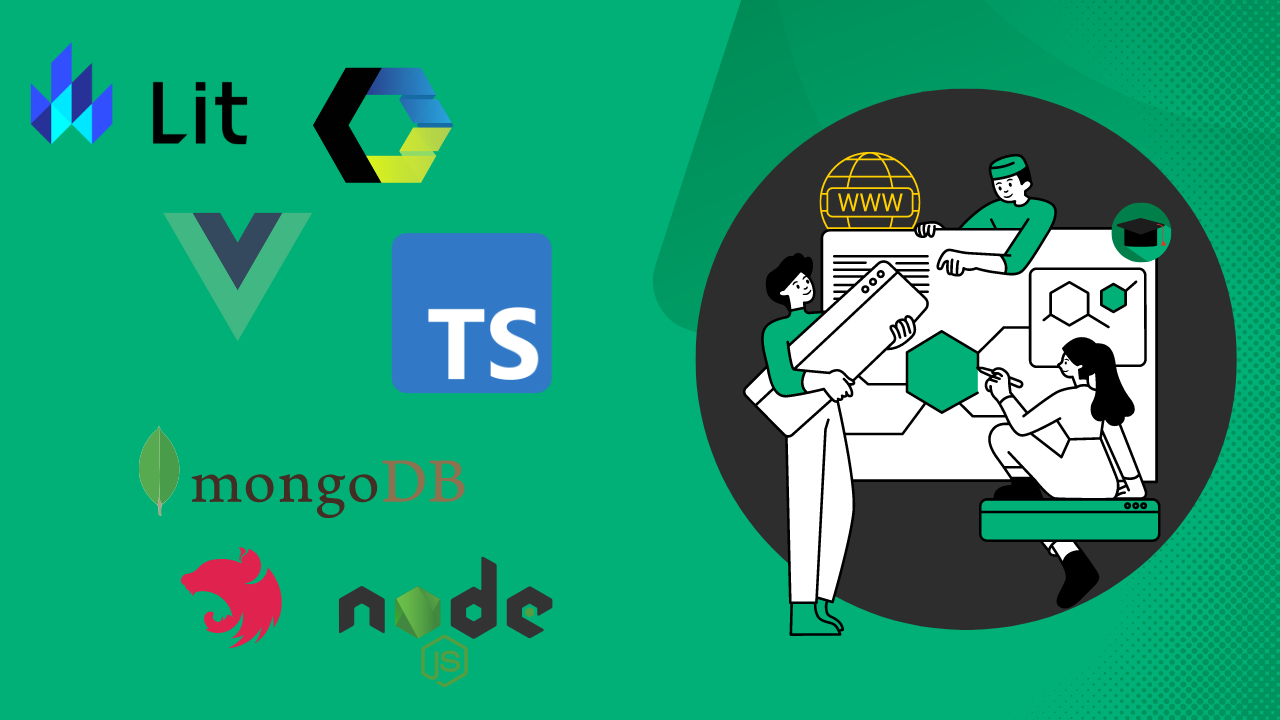
Purpose of React
While Web Components provide strong encapsulation for reusable components, React provides a declarative library that syncs the DOM with your data.
As a developer, you are free to use React in your Web Components, or to use Web Components in React, or both. — https://reactjs.org
The main difference between React components and Web Components is that React components can only be used inside a React application. A Web Component, on the other hand, can be used anywhere. We can use it in React, Vue, Angular, or any other web application.
React, or other frameworks are the way to go when your components need to handle a lot of data that needs to be passed down to child components.
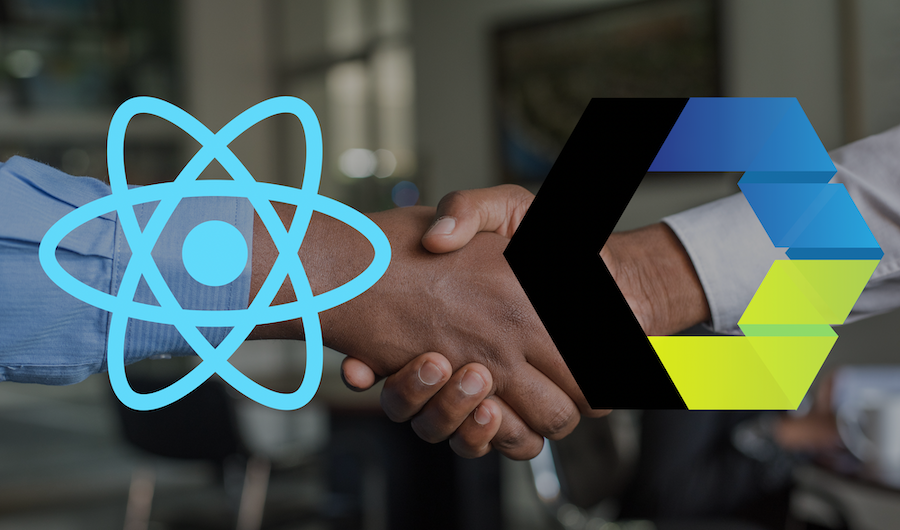
Building a Web Component
Let's go ahead and build our first Web Component, which we will integrate into a React application.
We will keep things simple and create an effortless custom element. If you are interested in building more complex Web Components, go through my series of articles: The Complete Web Component Guide.
Here is the JavaScript code for our hello-world
custom element:
Having this JavaScript code loaded in our HTML, we can easily include our component like this:
<hello-world></hello-world>
That will show our "Hello World!" headline. Try it yourself. Here is the CodePen.
Integrate a Web Component in React
Let's include our Web Component in a running React application. To make it easy, we will use a React starter project on Stackblitz. After that, we create the file hello-world.js
and put the code of our Web Component from above in it.
Next, we go into our React component and import our Javascript file, including the code of our Web Component:
import ./hello-world
And voila! The preview displays our Web Component. We successfully integrated a custom element into React.
Still, there is an error in the editor:
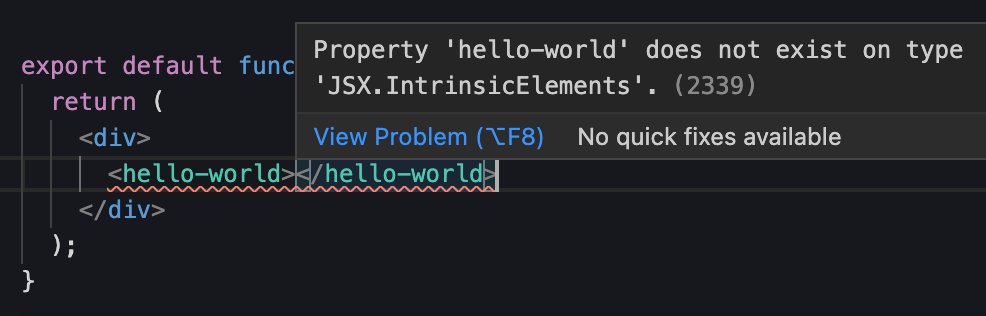
It says, "Property 'hello-world' does not exist on type 'JSX.IntrinsicElements'". The error occurs because React components should always start with an uppercase letter. Our component's name begins with a lowercase letter. So, should we change our custom tag to begin with an uppercase letter?
Let's try this:

As you can see, I changed our custom tag to Hello-world
. But, when calling customElements.define("Hello-world")
the browser throws an exception because we are violating one of two rules to be followed for the element's tag name:
- Name has to start with a lowercase ASCII character. It cannot have an uppercase ASCII character anywhere.
- Name must contain a dash — character. This dash character helps the browser to distinguish custom elements from regular elements.
We are only allowed to use lowercase characters, and the component needs to contain at least one dash. So, how to fix the error? Typescript gives us the possibility to declare our own types. To fix the error, we can define our custom element type by extending React's IntrinsicElements
interface like this:
Finally, we have successfully integrated our custom element to React without any errors. Here is the final Stackblitz of the example:
Final Thoughts
Using Web Components in React is easy. Building reusable components that are usable throughout frontend frameworks brings many benefits. We have learned that we should use Web Components when creating reusable components to use everywhere. When it comes to handling a lot of data, React is the way to go.
I hope you enjoyed reading this article. I am always happy to answer questions, and I am open to criticism. Feel free to contact me at any time 😊
Get in touch with me via LinkedIn or follow me on Twitter.
Looking for Productivity Browser Extensions?
At Tooltivity, we review the top productivity browser extensions. Read our detailed reviews here:
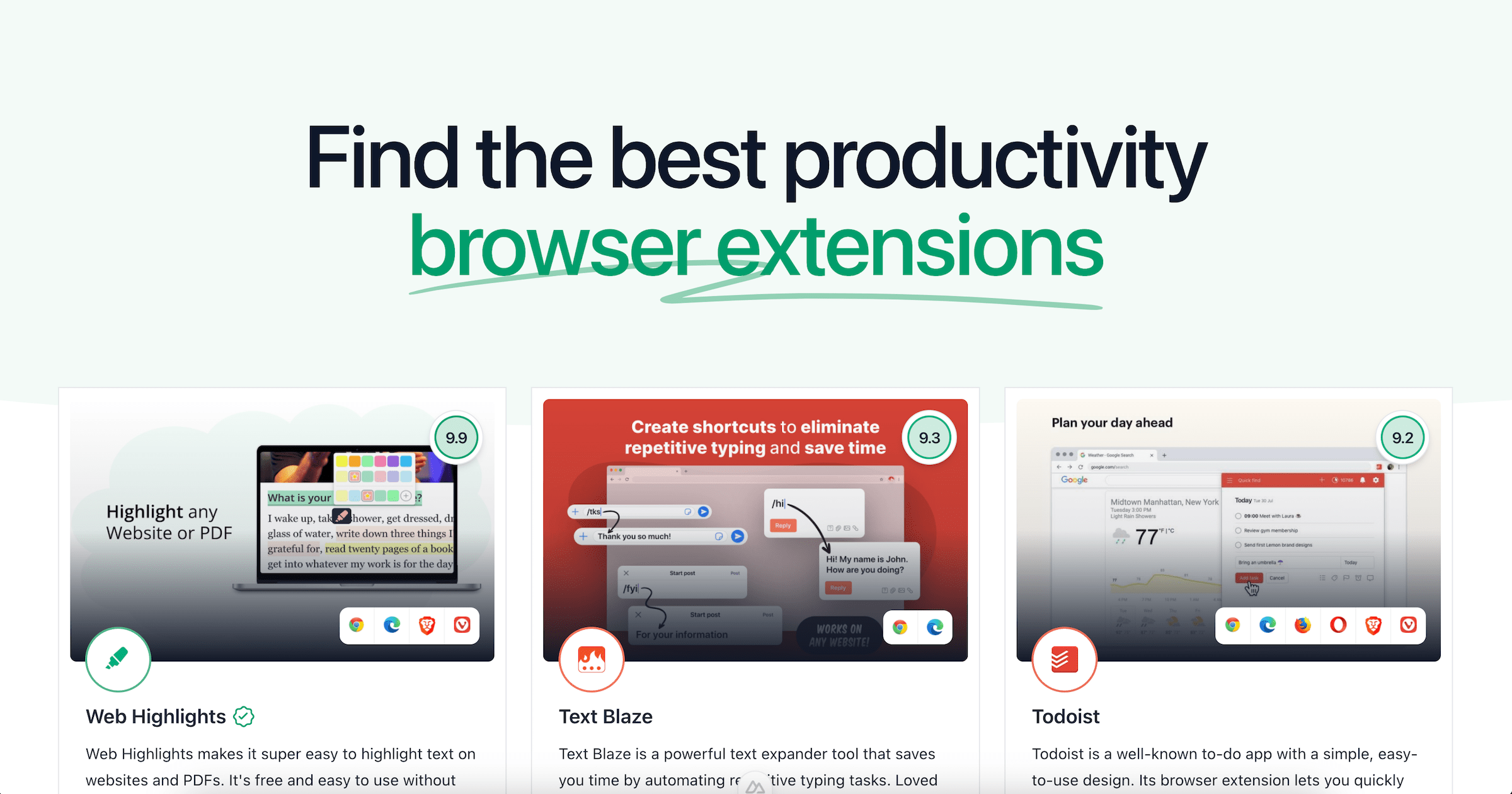