Build Your Own Custom Chrome History Extension with React - Part 1: Set Up
Step-by-Step Guide to Setting Up Your Custom Chrome History Extension in React. Learn how to create a Chrome extension with React and how to implement the Chrome History API
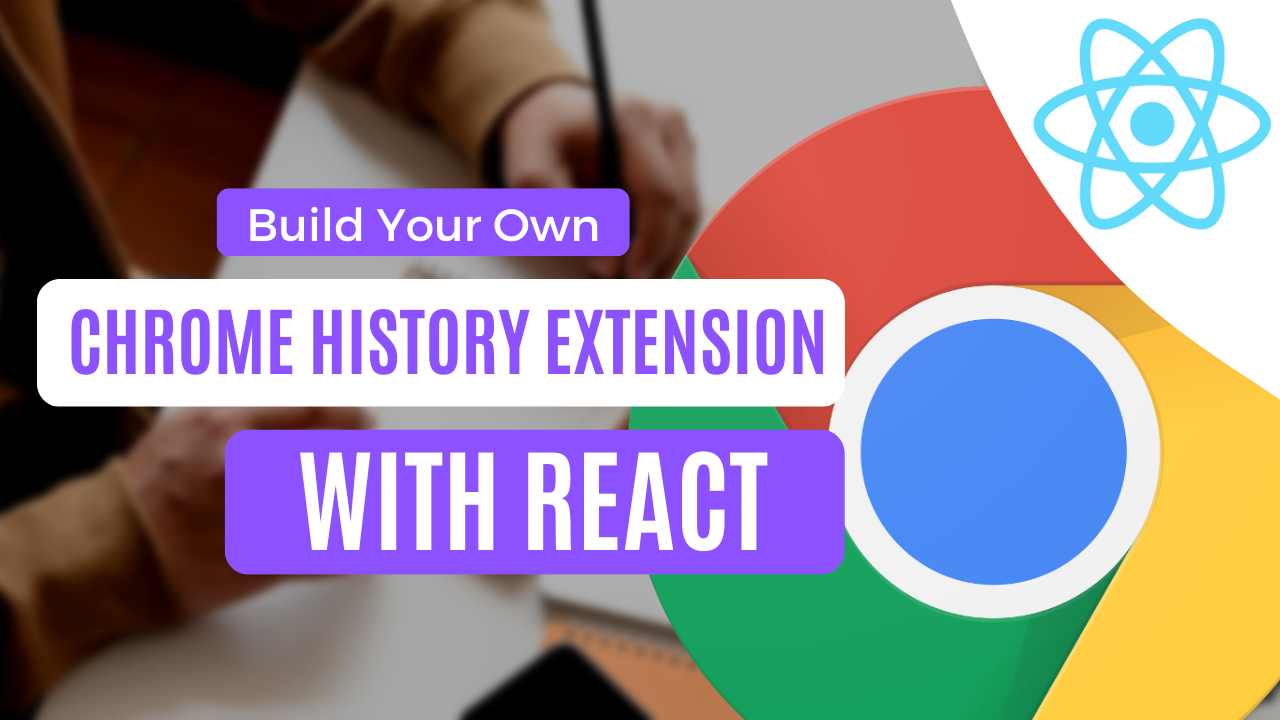
In this series of articles, I will walk you through creating your own browser history Chrome extension using React. We will use the Chrome History API to read our browser history and display it in our personalized React dashboard.
Here is what we are going to build:
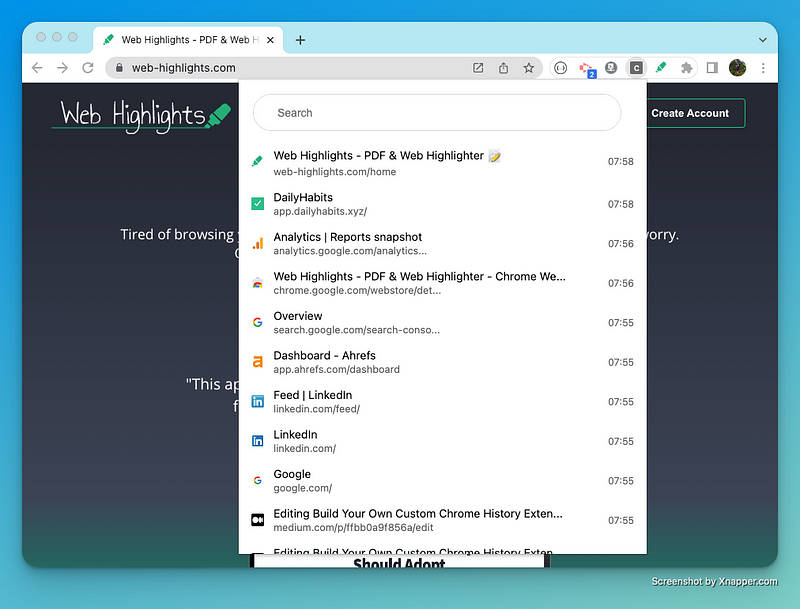
Without further ado, let’s get started.
Extension setup
Create an ‘/extension’ folder anywhere on your machine to get started. We will later copy & paste it into our React app. Then create a manifest.json
file inside our extension folder:
The manifest is our entry point, which defines our extension's metadata. For now, we only provide the name
, version
, and manifest_version
, which is enough to install the extension in our browser.
Open your Chrome browser and navigate to chrome://extensions.
Enable Developer Mode by clicking the toggle switch next to Developer mode. Click the “Load unpacked” button and select the extension directory of our repo containing ourmanifest.json
.
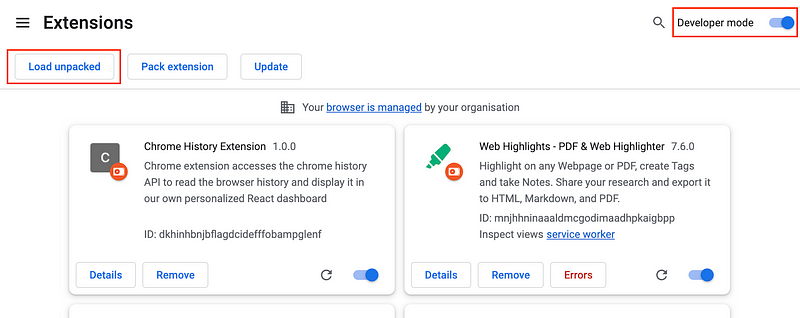
Congratulations! You just created a Chrome Extension! 👏
Currently, the extension doesn’t do anything. Therefore let’s define a page to open when clicking our extension icon in the chrome toolbar. We can do this by defining an action
in our manifest.json
.
An action’s popup will be shown when the user clicks on the extension’s action button in the toolbar. The popup can contain any HTML contents you like, and will be automatically sized to fit its contents. The popup cannot be smaller than 25x25 and cannot be larger than 800x600. - developer.chrome.com
Here is how to define an HTML popup that opens when clicking our extension icon:
Our index.html
file contains a simple HTML file with some styles to set the width and height of the body element. Furthermore, it provides the JavaScript for our React app by loading the app.js
file.
As we still need to set up the React app, our popup only contains a blank page with the defined width and height. You should now be able to see it when clicking the extension icon:
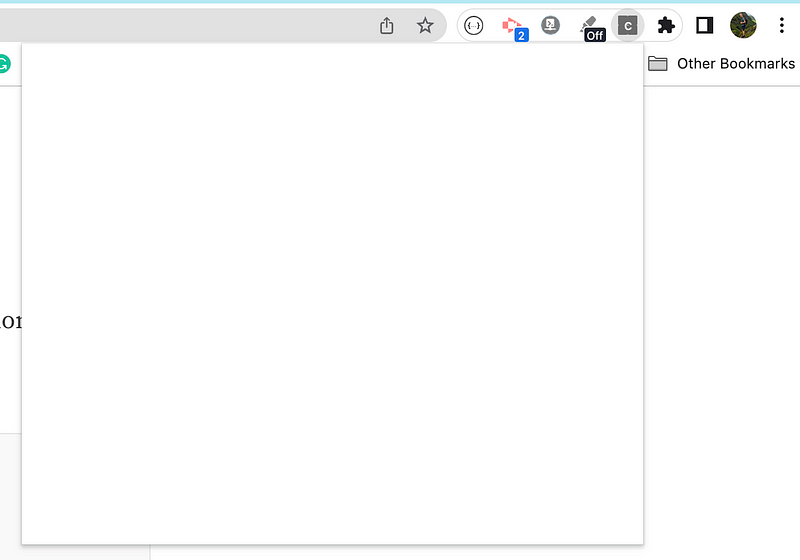
If you like, you can also include a custom icon for your extension by providing the default_icon
attribute:
React Setup
We will build a React application. Therefore let us create some boilerplate by running the following script using the create-react-app
CLI:
npx create-react-app chrome-history-extension --template typescript
Let’s eliminate all the unnecessary stuff. Remove all CSS styles and the React template code in the App.tsx
component. You can also delete the /public folder (We will replace it with our /extension folder later).
To test our setup, let’s put a simple “Hello from React App 👋” headline to our component:
Finally, we will adjust our index.tsx
file a bit to create the entry point of our application programmatically:
Webpack setup
In the next step, we will configure an application bundler to build our chrome extension automatically. Furthermore, we will set up a development environment to test our extension.
We will use Webpack 5 as a module bundler. To use it, we install webpack and the copy-webpack-plugin plugin by running:
yarn add -D webpack@^5 copy-webpack-plugin
OR
npm i -D webpack@5 copy-webpack-plugin
Now, let’s create a webpack.config.js
file and put the following code in it:
If you don’t know how webpack works, I highly recommend learning it. Still, for now, it’s relevant to know that it will replace the built-in react-scripts
configuration to start and build the app. Therefore it uses a ts-loader
to transpile our Typescript .tsx
templates into JavaScript and bundles them into one Javascript file (app.js
).
Additionally, we will create a CopyPlugin
that transfers all files from the /extension directory, including the manifest.json
file, to the /dist build folder.
Navigate to the package.json
file and look at our "start"
and "build"
scripts. They should look like this:
Let’s replace those scripts with webpack:
You can see that our build
script simply calls webpack
. By default, webpack will look for a webpack.config.js
configuration file that we just created.
Furthermore, we defined a start
script. This script takes a different webpack configuration with a dev server, enabling us to test our extension locally without installing the extension. By that, we can better and faster test our extension. Here is what the development configuration looks like:
This config takes our original configuration from the webpack.config.js
file and overrides some values. We configure a webpack dev server to serve our application statically from the extension
folder on port 8080.
Now, you should be able to run yarn start
and see our application running on localhost:8080.
Furthermore, you can run yarn build
, and webpack should automatically generate all necessary files for our extension and copy them to the /dist folder. We can now load our unpacked extension from this folder.
Opening our popup should show the React app containing our “Hello From React App 👋” headline:
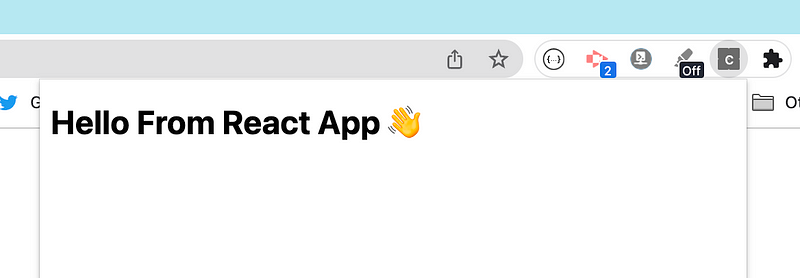
We are finished with the setup and will continue implementing the chrome history API in the following article. Here is the Github repository, including the extension's final version.
Final Thoughts
In this article, we laid the groundwork for displaying our Chrome history in our React Chrome extension in subsequent articles. We’ve covered the essential steps for setting up a Chrome extension and integrating React with Webpack to bundle them into a Chrome extension effortlessly.
With these building blocks in place, we’re now ready for the next article, in which we will learn how to access the Chrome History API to show it inside our extension. Follow me or subscribe to get my stories via email not to miss the next article.
I hope you enjoyed reading this article. I am always happy to answer questions and am open to criticism. Feel free to contact me at any time! Get in touch with me via LinkedIn, Twitter, or leave a comment.